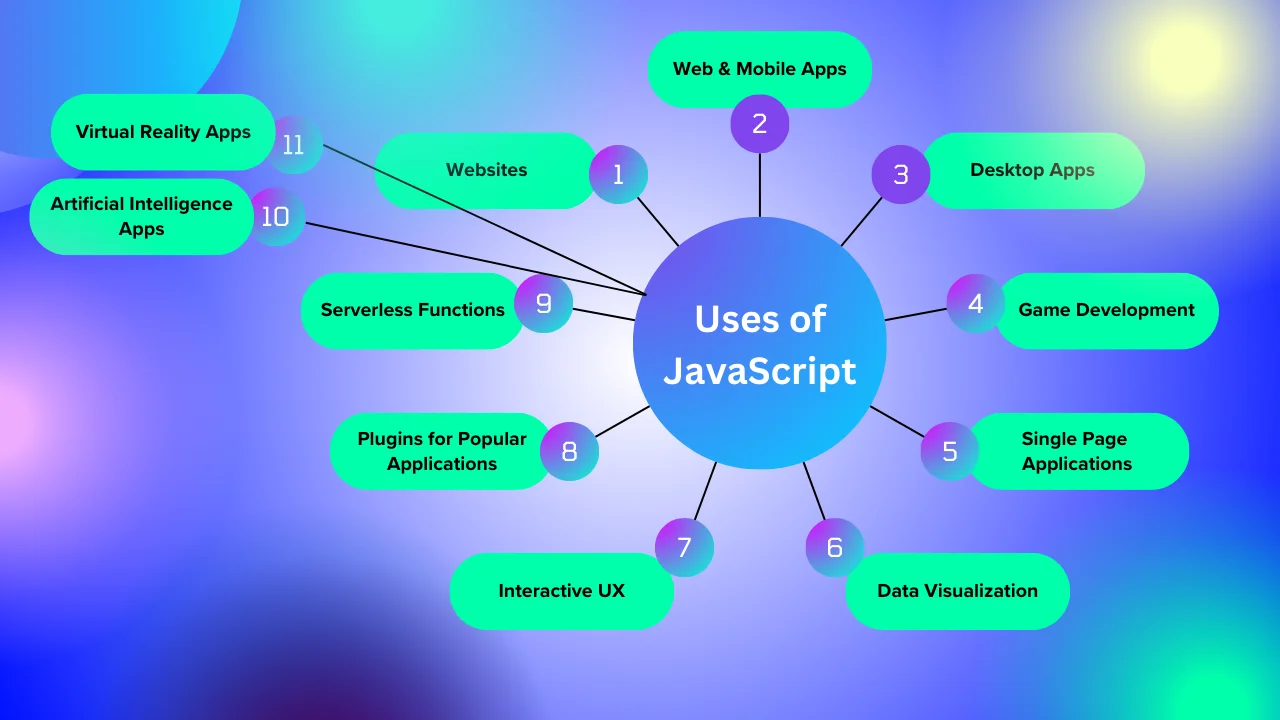
JavaScript: A Complete Guide to Understanding and Mastery
What is JavaScript?
JavaScript is a high-level, versatile programming language primarily used for building interactive and dynamic features on websites. Created in 1995 by Brendan Eich at Netscape, it has become a core technology of the web alongside HTML and CSS. Unlike static languages, JavaScript runs in web browsers and enables features like dynamic content updates, form validation, animations, and user interaction without needing to reload the page. It uses an event-driven, prototype-based model and supports various paradigms such as functional, object-oriented, and imperative programming.JavaScript can also run server-side through environments like Node.js, making it a powerful full-stack development tool. Its features include manipulating the DOM (Document Object Model) for customizing webpage content, handling asynchronous operations with promises and async/await, and integrating APIs for real-time data communication. Beyond websites, it is widely used in mobile app development, game design, and machine learning, showcasing its adaptability. As an open standard, JavaScript is constantly evolving, ensuring its relevance across industries and applications.
Why JavaScript Matters
JavaScript stands out because of its versatility and ubiquity. Originally intended for client-side scripting in web browsers, it has expanded to encompass a wide range of use cases, from server-side development (Node.js) to mobile applications, desktop applications, and even IoT devices. It is a multi-paradigm language, supporting imperative, functional, and object-oriented programming styles, making it adaptable to a variety of development needs.What is a Website and the Role of JavaScript in Web Development
A website is a digital platform consisting of interconnected web pages and resources, hosted on a server and accessible via the internet using a web browser. It serves as a medium for sharing information, offering services, or enabling interaction.
JavaScript is a fundamental technology used in websites to create interactivity and dynamic behavior. It allows for real-time updates, user engagement through event handling, and seamless interaction between the user and the platform. JavaScript enables features like dynamic content loading, responsive design adjustments, and asynchronous communication with servers, enhancing user experience. It plays a crucial role in modern web development, powering advanced frameworks and tools that make websites functional, efficient, and user-friendly.
// Select the DOM element to display the greetingconstgreetingElement=document.querySelector('.greeting'); /**
* Function to determine an appropriate greeting
* based on the current time.
* * @returns{string} A greeting message ('Good Morning', 'Good Afternoon', or 'Good Evening')
*/
functiongetGreeting(){
constcurrentHour=newDate().getHours(); // Get the current hour (0–23)// Determine the greeting messageif(currentHour< 12){
return'Good Morning!'} else if(currentHour18){
return'Good Afternoon!'} else{
return'Good Evening!'}} // Update the content of the selected DOM element with the greeting
greetingElement.textContent=getGreeting();
Web & Mobile Apps
JavaScript is one of the most versatile programming languages in modern development, enabling the creation of interactive and scalable applications for both web and mobile platforms. Its ecosystem provides robust tools for frontend, backend, and cross-platform development, making it indispensable in the software development landscape.
import{ useState, useEffect}from'react'; constWeatherApp=()=>{
const[weather, setWeather]=useState(null);
const[city, setCity]=useState('New York'); useEffect(()=>{
constfetchWeather=async()=>{
constresponse=awaitfetch(`https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=YOUR_API_KEY`);
constdata=awaitresponse.json();
setWeather(data)}; fetchWeather()}, [city]); return(
<div><h1>Weather App</h1><inputtype="text"placeholder="Enter city"value={city}onChange={(e)=>setCity(e.target.value)}/>{weather&& (
<div><h2>{weather.name}</h2><p>{weather.weather[0].description}</p><p>{Math.round(weather.main.temp- 273.15)}°C</p></div>)}
</div>)}; export defaultWeatherApp;
constexpress=require('express');
constapp=express();
constport=3000; app.use(express.json()); lettasks=[
{ id: 1, name: 'Learn JavaScript', completed: false},
{ id: 2, name: 'Build a web app', completed: true},
]; // Get all tasksapp.get('/tasks', (req, res)=>{
res.json(tasks)}); // Add a new taskapp.post('/tasks', (req, res)=>{
constnewTask={ id: tasks.length+ 1, ...req.body};
tasks.push(newTask);
res.status(201).json(newTask)}); // Update a taskapp.put('/tasks/:id', (req, res)=>{
consttaskId=parseInt(req.params.id);
consttaskIndex=tasks.findIndex((task)=>task.id===taskId); if(taskIndex===-1) returnres.status(404).send('Task not found'); tasks[taskIndex]={ id: taskId, ...req.body};
res.json(tasks[taskIndex])}); // Delete a taskapp.delete('/tasks/:id', (req, res)=>{
tasks=tasks.filter((task)=>task.id!==parseInt(req.params.id));
res.status(204).send()}); app.listen(port, ()=>{
console.log(`Server running on http://localhost:${port}`)});
import{ useState}from'react';
import{ View, Text, TextInput, Button, FlatList, StyleSheet}from'react-native'; constToDoApp=()=>{
const[task, setTask]=useState('');
const[tasks, setTasks]=useState([]); constaddTask=()=>{
if(task){
setTasks([
...tasks,{ key: Date.now().toString(), value: task}
]);
setTask('')}}; return(
<Viewstyle={styles.container}><Textstyle={styles.title}>To-Do App
</Text><TextInputstyle={styles.input}placeholder="Enter task"value={task}onChangeText={setTask}/><Buttontitle="Add Task"onPress={addTask}/><FlatListdata={tasks}renderItem={({ item})=><Textstyle={styles.task}>{item.value}
</Text>}/></View>)}; conststyles=StyleSheet.create({
container:{ flex: 1, padding: 20, backgroundColor: '#fff'},
title:{ fontSize: 24, fontWeight: 'bold', marginBottom: 20},
input:{ borderBottomWidth: 1, marginBottom: 20, padding: 8},
task:{ fontSize: 18, padding: 8, borderBottomWidth: 1, borderBottomColor: '#ccc'},}); export defaultToDoApp;
constexpress=require('express');
consthttp=require('http');
const{ Server}=require('socket.io'); constapp=express();
constserver=http.createServer(app);
constio=newServer(server); app.get('/', (req, res)=>{
res.sendFile(__dirname+ '/index.html')}); io.on('connection', (socket)=>{
console.log('A user connected'); socket.on('chat message', (msg)=>{
io.emit('chat message', msg)}); socket.on('disconnect', ()=>{
console.log('A user disconnected')})}); server.listen(3000, ()=>{
console.log('Server running on http://localhost:3000')});
<!DOCTYPE html><html><head><title>Chat App</title></head><body><h1>Chat App</h1><ulid="messages"></ul><formid="form"action=""><inputid="input"autocomplete="off"/><button>Send</button></form><scriptsrc="/socket.io/socket.io.js"></script><script>constsocket=io();
constform=document.getElementById('form');
constinput=document.getElementById('input');
constmessages=document.getElementById('messages'); form.addEventListener('submit', (e)=>{
e.preventDefault();
if(input.value){
socket.emit('chat message', input.value);
input.value=''}}); socket.on('chat message', (msg)=>{
constitem=document.createElement('li');
item.textContent=msg;
messages.appendChild(item)});
</script></body></html>
Desktop Apps
JavaScript has emerged as a powerful language for creating desktop applications through modern frameworks and runtime environments. By combining JavaScript with web technologies like HTML and CSS, developers can build cross-platform desktop applications that function seamlessly on Windows, macOS, and Linux. These apps leverage frameworks to access native system features such as file systems, hardware integrations, and operating system-level functionalities, offering the performance and look of traditional native applications.
// Importing the Electron moduleconst{ app, BrowserWindow}=require('electron'); letmainWindow; // Function to create the main windowfunctioncreateMainWindow(){
mainWindow=newBrowserWindow({
width: 800, // Window widthheight: 600, // Window heightwebPreferences:{
nodeIntegration: true, // Enable Node.js integrationcontextIsolation: false, // Disable context isolation (if required)},}); // Load the HTML file into the windowmainWindow.loadFile('index.html'); // Optional: Open DevTools for debugging// mainWindow.webContents.openDevTools();// Handle the window's close eventmainWindow.on('closed', ()=>{
mainWindow=null})} // Event: App is ready to startapp.whenReady().then(()=>{
createMainWindow(); // MacOS-specific behavior: Reopen the app when no windows are openapp.on('activate', ()=>{
if(BrowserWindow.getAllWindows().length===0) createMainWindow()})}); // Event: All windows are closedapp.on('window-all-closed', ()=>{
// Close the app entirely on non-Mac platformsif(process.platform!=='darwin') app.quit()});
Game Development
JavaScript is a prominent language for game development, especially for browser-based games and lightweight applications. Its seamless integration with HTML5 and WebGL enables developers to create 2D and 3D games that run directly in web browsers without additional plugins. Using the HTML5 <canvas> element, developers can render graphics, animations, and real-time interactions, while libraries like Three.js and PixiJS streamline the development of 3D and 2D games, respectively. Frameworks such as Phaser.js offer robust tools for building interactive games with physics engines, animations, and asset management. JavaScript's versatility and wide browser compatibility make it a go-to choice for cross-platform game development, ranging from simple arcade games to more complex graphical experiences.
// Phaser Game Configurationconstconfig={
type: Phaser.AUTO,
width: 800,
height: 600,
physics:{
default: 'arcade',
arcade:{
gravity:{ y: 300},
debug: false,},},
scene:{
preload: preload,
create: create,
update: update,},}; // Initialize Phaser Gameconstgame=newPhaser.Game(config); // Game Variablesletplayer;
letstars;
letbombs;
letcursors;
letscore=0;
letscoreText; /**
* Preload assets required for the game.
*/functionpreload(){
this.load.image('sky', 'https://labs.phaser.io/assets/skies/space3.png');
this.load.image('ground', 'https://labs.phaser.io/assets/platforms/platform.png');
this.load.image('star', 'https://labs.phaser.io/assets/sprites/star.png');
this.load.image('bomb', 'https://labs.phaser.io/assets/sprites/bomb.png');
this.load.spritesheet('dude', 'https://labs.phaser.io/assets/sprites/dude.png',{
frameWidth: 32,
frameHeight: 48,})} /**
* Create game objects, animations, and logic.
*/functioncreate(){
// Add backgroundthis.add.image(400, 300, 'sky'); // Create static platformsconstplatforms=this.physics.add.staticGroup();
platforms.create(400, 568, 'ground').setScale(2).refreshBody();
platforms.create(600, 400, 'ground');
platforms.create(50, 250, 'ground');
platforms.create(750, 220, 'ground'); // Create player characterplayer=this.physics.add.sprite(100, 450, 'dude');
player.setBounce(0.2);
player.setCollideWorldBounds(true); // Create player animationscreatePlayerAnimations(this); // Enable player-platform collisionthis.physics.add.collider(player, platforms); // Add collectible starsstars=createStars(this, platforms); // Add bombs (obstacles)bombs=this.physics.add.group();
this.physics.add.collider(bombs, platforms);
this.physics.add.collider(player, bombs, hitBomb, null, this); // Display scorescoreText=this.add.text(16, 16, 'Score: 0',{ fontSize: '32px', fill: '#fff'}); // Add keyboard controlscursors=this.input.keyboard.createCursorKeys()} /**
* Update game loop (runs continuously).
*/functionupdate(){
handlePlayerMovement()} /**
* Creates player animations.
* @param{object} scene - Current game scene.
*/functioncreatePlayerAnimations(scene){
scene.anims.create({
key: 'left',
frames: scene.anims.generateFrameNumbers('dude',{ start: 0, end: 3}),
frameRate: 10,
repeat: -1,}); scene.anims.create({
key: 'turn',
frames: [{ key: 'dude', frame: 4}],
frameRate: 20,}); scene.anims.create({
key: 'right',
frames: scene.anims.generateFrameNumbers('dude',{ start: 5, end: 8}),
frameRate: 10,
repeat: -1,})} /**
* Handles player movement based on keyboard input.
*/functionhandlePlayerMovement(){
if(cursors.left.isDown){
player.setVelocityX(-160);
player.anims.play('left', true)} else if(cursors.right.isDown){
player.setVelocityX(160);
player.anims.play('right', true)} else{
player.setVelocityX(0);
player.anims.play('turn')} if(cursors.up.isDown&& player.body.touching.down){
player.setVelocityY(-330)}} /**
* Collects a star and updates the score.
* @param{object} player - Player character.
* @param{object} star - Star object.
*/functioncollectStar(player, star){
star.disableBody(true, true);
score+=10;
scoreText.setText(`Score: ${score}`); // Check if all stars are collectedif(stars.countActive(true)===0){
stars.children.iterate((child)=>{
child.enableBody(true, child.x, 0, true, true)}); // Spawn a bombconstx=player.x< 400? Phaser.Math.Between(400, 800) : Phaser.Math.Between(0, 400); constbomb=bombs.create(x, 16, 'bomb');
bomb.setBounce(1);
bomb.setCollideWorldBounds(true);
bomb.setVelocity(Phaser.Math.Between(-200, 200), 20)}} /**
* Handles player collision with a bomb (game over).
* @param{object} player - Player character.
* @param{object} bomb - Bomb object.
*/functionhitBomb(player, bomb){
this.physics.pause();
player.setTint(0xff0000);
player.anims.play('turn');
scoreText.setText('Game Over!')}
Single Web Application
A Single Page Application (SPA) is a web application designed to provide a dynamic, seamless user experience by loading a single HTML page and dynamically updating its content using JavaScript. Unlike traditional multi-page applications (MPAs) that reload the entire page for every user interaction, SPAs fetch only the required data from the server and update the view in real-time without a full-page reload. SPAs are built to enhance performance and improve user engagement by minimizing page load times and providing a fluid, app-like experience. They rely on technologies such as JavaScript for DOM manipulation, asynchronous data fetching through APIs, and routing to manage navigation within the application. This architecture enables a more interactive interface, faster response times, and reduced server load. Despite their advantages, SPAs come with challenges, including SEO optimization due to their reliance on JavaScript for rendering content. To address this, developers often implement server-side rendering (SSR) or pre-rendering techniques. SPAs also require careful management of application state and may have a slightly higher initial load time due to the need to download all essential resources upfront. However, techniques like code splitting, caching, and lazy loading can mitigate these issues effectively.
// Select all links with data-link attributeconstlinks=document.querySelectorAll('[data-link]');
constsections=document.querySelectorAll('.content'); // Add event listeners to linkslinks.forEach(link=>{
link.addEventListener('click', (e)=>{
e.preventDefault(); // Hide all sectionssections.forEach(section=>section.classList.add('hidden')); // Show the target sectionconsttarget=link.getAttribute('href').substring(1);
document.getElementById(target).classList.remove('hidden'); // Update browser historyhistory.pushState(null, '', `#${target}`)})}); // Handle browser back/forward navigationwindow.addEventListener('popstate', ()=>{
consthash=location.hash.substring(1) || 'home';
sections.forEach(section=>section.classList.add('hidden'));
document.getElementById(hash).classList.remove('hidden')}); // Set the default section based on URL hashconstinitialHash=location.hash.substring(1) || 'home';
document.getElementById(initialHash).classList.remove('hidden');
Data Visualization
Data Visualization in JavaScript involves transforming raw datasets into graphical representations to enable better understanding, analysis, and communication of information. By using JavaScript, developers can create dynamic, interactive, and visually appealing visuals that are accessible across multiple platforms. It plays a crucial role in decision-making by simplifying complex data and highlighting trends or insights.
// Create a bar chart for sales dataconstctx=document.getElementById('salesChart').getContext('2d');
constsalesChart=newChart(ctx,{
type: 'bar',
data:{
labels: ['January', 'February', 'March', 'April'],
datasets: [{
label: 'Revenue (in $)',
data: [15000, 20000, 18000, 22000],
backgroundColor: [
'rgba(75, 192, 192, 0.5)',
'rgba(54, 162, 235, 0.5)',
'rgba(255, 206, 86, 0.5)',
'rgba(153, 102, 255, 0.5)'],
borderColor: [
'rgba(75, 192, 192, 1)',
'rgba(54, 162, 235, 1)',
'rgba(255, 206, 86, 1)',
'rgba(153, 102, 255, 1)'],
borderWidth: 1}]},
options:{
responsive: true,
plugins:{
tooltip:{
enabled: true}},
scales:{
y:{
beginAtZero: true}}}});
Interactive UX
Interactive UX (User Experience) in JavaScript refers to the integration of dynamic and engaging functionalities within a web application to enhance user interaction and satisfaction. It focuses on creating responsive, adaptive, and seamless interfaces that react to user actions, improving the overall usability and accessibility of a website or application. JavaScript plays a pivotal role in crafting these interactive experiences, bridging the gap between static designs and dynamic, user-centric solutions.
// Modal Functionalityconstmodal=document.querySelector('.modal');
constopenModalButton=document.querySelector('.open-modal');
constcloseModalButton=document.querySelector('.close-modal'); openModalButton.addEventListener('click', ()=>{
modal.style.display='block'; // Show modalmodal.classList.add('fade-in'); // Add animation class}); closeModalButton.addEventListener('click', ()=>{
modal.style.display='none'; // Hide modalmodal.classList.remove('fade-in'); // Remove animation class}); // Form Validationconstform=document.querySelector('.form');
constnameInput=document.querySelector('#name');
constemailInput=document.querySelector('#email');
constnameError=document.querySelector('.name-error');
constemailError=document.querySelector('.email-error'); form.addEventListener('submit', (event)=>{
letisValid=true; // Validate Nameif(nameInput.value.trim()===''){
nameError.textContent='Name is required.';
isValid=false} else{
nameError.textContent=''} // Validate Emailif(!emailInput.value.match(/^\S+@\S+\.\S+$/)){
emailError.textContent='Please enter a valid email address.';
isValid=false} else{
emailError.textContent=''} // Prevent Form Submission if Invalidif(!isValid) event.preventDefault()}); // Button Hover EffectconsthoverButton=document.querySelector('.hover-button'); hoverButton.addEventListener('mouseenter', ()=>{
hoverButton.style.transform='scale(1.1)';
hoverButton.style.transition='transform 0.3s ease'}); hoverButton.addEventListener('mouseleave', ()=>{
hoverButton.style.transform='scale(1)'});
Plugins for Popular Applications
JavaScript plugins are reusable pieces of code that add specific features or extend the functionality of an application, website, or software. These plugins integrate seamlessly with popular JavaScript frameworks or libraries and are often used to enhance user experience, simplify development, or improve application efficiency.
(function(){/**
* Tooltip Plugin
* This plugin adds custom tooltip functionality to elements with the `data-tooltip` attribute.
* Usage: Add `data-tooltip="Your tooltip text"` to any HTML element.
* Example: <div data-tooltip="Hello, I am a tooltip!"></div>
*/classTooltip{constructor(){this.tooltipElement=null; // Tooltip containerthis.offset=8; // Space between the tooltip and the target elementthis.initialize();
}/**
* Initialize the Tooltip Plugin
* Attaches event listeners to elements with the `data-tooltip` attribute.
*/initialize(){constelements=document.querySelectorAll("[data-tooltip]");
elements.forEach((element) =>{element.addEventListener("mouseenter", (event) =>this.showTooltip(event));
element.addEventListener("mouseleave", () =>this.hideTooltip());
});
}/**
* Show the tooltip for the hovered element.
* @param{MouseEvent} event - The mouse event triggered on hover.
*/showTooltip(event) {consttarget=event.currentTarget;
consttooltipText=target.getAttribute("data-tooltip"); if(!tooltipText)return; // Create the tooltip elementthis.tooltipElement=document.createElement("div");
this.tooltipElement.className="custom-tooltip";
this.tooltipElement.textContent=tooltipText; document.body.appendChild(this.tooltipElement); // Position the tooltipconst{top, left, width, height }=target.getBoundingClientRect();
consttooltipHeight=this.tooltipElement.offsetHeight;
consttooltipWidth=this.tooltipElement.offsetWidth; this.tooltipElement.style.top=`${top - tooltipHeight - this.offset}px`;
this.tooltipElement.style.left=`${left + width / 2- tooltipWidth / 2}px`;
}/**
* Hide and remove the tooltip element.
*/hideTooltip(){if(this.tooltipElement){this.tooltipElement.remove();
this.tooltipElement=null;
}}}// Initialize the plugin when the DOM is fully loadeddocument.addEventListener("DOMContentLoaded", () =>{newTooltip();
});
})();
Serverless Function
Serverless Functions in JavaScript are lightweight, event-driven pieces of code that run on cloud platforms without requiring developers to manage the underlying server infrastructure. These functions are designed to execute specific tasks and are hosted in a serverless architecture, where the cloud provider handles server provisioning, scaling, and maintenance. Despite the name, "serverless" does not mean the absence of servers; it means the servers are abstracted away, allowing developers to focus solely on writing application logic.
// AWS Lambda Function (index.js)exports.handler=async(event) =>{try{// Extract the 'name' query parameter; default to 'World' if not providedconstuserName=event.queryStringParameters?.name || "World"; // Build the response payloadconstresponsePayload={message: `Hello, ${userName}! Welcome to the serverless function.`,
timestamp: newDate().toISOString(),
}; // Return a successful HTTP responsereturn{statusCode: 200,
headers: {"Content-Type": "application/json",
},
body: JSON.stringify(responsePayload),
};
}catch(error) {console.error("Error occurred in the Lambda function:", error); // Return a generic error responsereturn{statusCode: 500,
headers: {"Content-Type": "application/json",
},
body: JSON.stringify({message: "Internal Server Error: Unable to process the request.",
details: error.message,
}),
};
}};
Artificial Intelligence Apps
Artificial Intelligence (AI) apps in JavaScript are applications or systems built using JavaScript to perform tasks that typically require human intelligence. These tasks include understanding language, recognizing images, making decisions, or predicting outcomes. AI apps leverage machine learning (ML), natural language processing (NLP), computer vision, and other AI techniques.JavaScript, being the most widely used language for web development, enables developers to integrate AI capabilities directly into web and mobile applications. Using libraries and frameworks, developers can create intelligent and interactive user experiences.
// Import TensorFlow.jsimport* astf from'@tensorflow/tfjs'; // Select DOM ElementsconstimageInput=document.getElementById('imageInput');
constimageCanvas=document.getElementById('imageCanvas');
constclassifyBtn=document.getElementById('classifyBtn');
constresult=document.getElementById('result'); // Class Labels for MobileNetconstclassLabels=[
"Class 1: Example Label", "Class 2: Example Label", // Update with actual MobileNet class labels// Continue for all classes supported by MobileNet]; // Load Pre-trained Model (MobileNet)letmodel;
constloadModel=async() =>{try{model=awaittf.loadLayersModel('https://storage.googleapis.com/tfjs-models/tfjs/mobilenet_v1_0.25_224/model.json');
console.log('Model Loaded Successfully');
classifyBtn.disabled=false; // Enable the classify button}catch(error) {console.error('Error loading model:', error);
result.textContent='Failed to load the AI model. Please check your internet connection.';
}}; // Handle Image UploadimageInput.addEventListener('change', (event) =>{constfile=event.target.files[0];
if(!file) {result.textContent='No file selected. Please upload an image.';
return;
}constreader=newFileReader();
reader.onload=() =>{constimg=newImage();
img.onload=() =>{constctx=imageCanvas.getContext('2d');
imageCanvas.width=224;
imageCanvas.height=224;
ctx.drawImage(img, 0, 0, 224, 224); // Resize image to 224x224 for model inputresult.textContent='Image uploaded successfully. Click "Classify" to analyze.';
};
img.src=reader.result;
};
reader.readAsDataURL(file);
}); // Classify ImageclassifyBtn.addEventListener('click', async() =>{if(!model) {result.textContent='Model not loaded yet. Please wait.';
return;
}try{constctx=imageCanvas.getContext('2d');
constimageData=ctx.getImageData(0, 0, 224, 224); // Get image data from canvasconsttensor=tf.browser.fromPixels(imageData)
.toFloat()
.div(tf.scalar(127.5))
.sub(tf.scalar(1))
.expandDims(); // Normalize and reshape image dataconstpredictions=model.predict(tensor).dataSync(); // Predict image class probabilitiesconsttopPredictionIndex=predictions.indexOf(Math.max(...predictions)); // Display Results with Confidence Scoreconstconfidence=(predictions[topPredictionIndex] * 100).toFixed(2);
constpredictedLabel=classLabels[topPredictionIndex] || `Unknown Class (${topPredictionIndex})`; result.textContent=`Prediction: ${predictedLabel} (Confidence: ${confidence}%)`;
}catch(error) {console.error('Error during prediction:', error);
result.textContent='An error occurred while classifying the image. Please try again.';
}}); // Initialize ModelloadModel();
Virtual Reality Apps
Virtual Reality (VR) apps in JavaScript refer to immersive applications that allow users to interact with 3D environments or virtual worlds using devices like VR headsets (e.g., Oculus, HTC Vive) or directly in a browser. JavaScript enables developers to build VR apps that run seamlessly on the web without requiring additional software installation.By leveraging WebVR, WebXR, and libraries like Three.js or A-Frame, developers can create dynamic, interactive VR experiences for gaming, education, architecture, training simulations, and more.